Create SOAP Web Service in Java using Eclipse
In this tutorial, we will build a web service using SOAP.
Step 1 : Create a dynamic web project in eclipse/STS
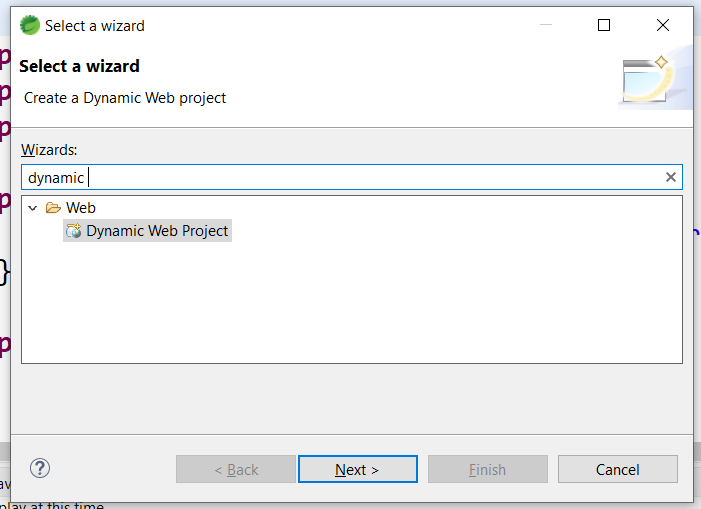
Step 2 : Fill in the deails as below
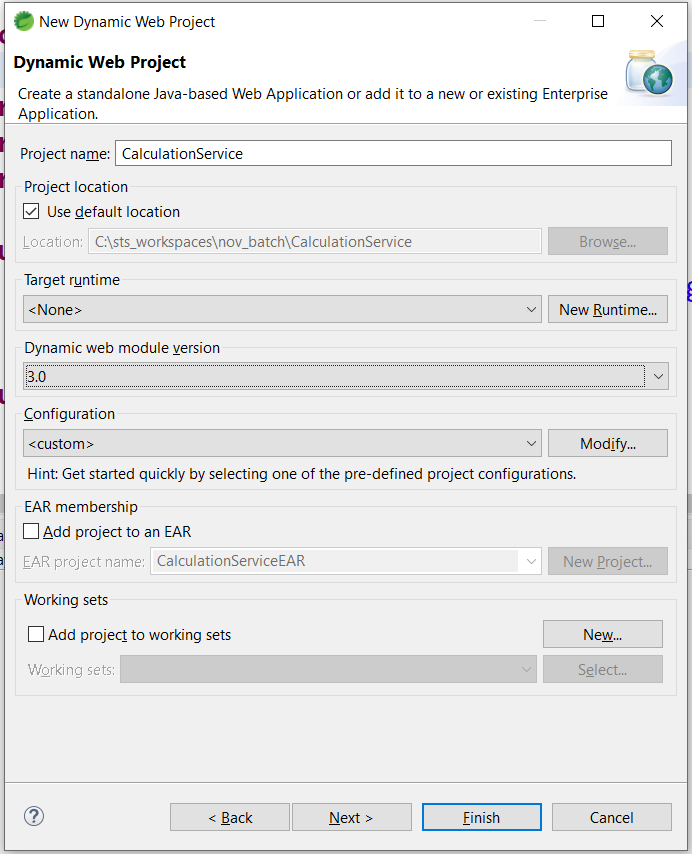
Step 3 : Click Next. Don’t forget to click the check boxes as shown below
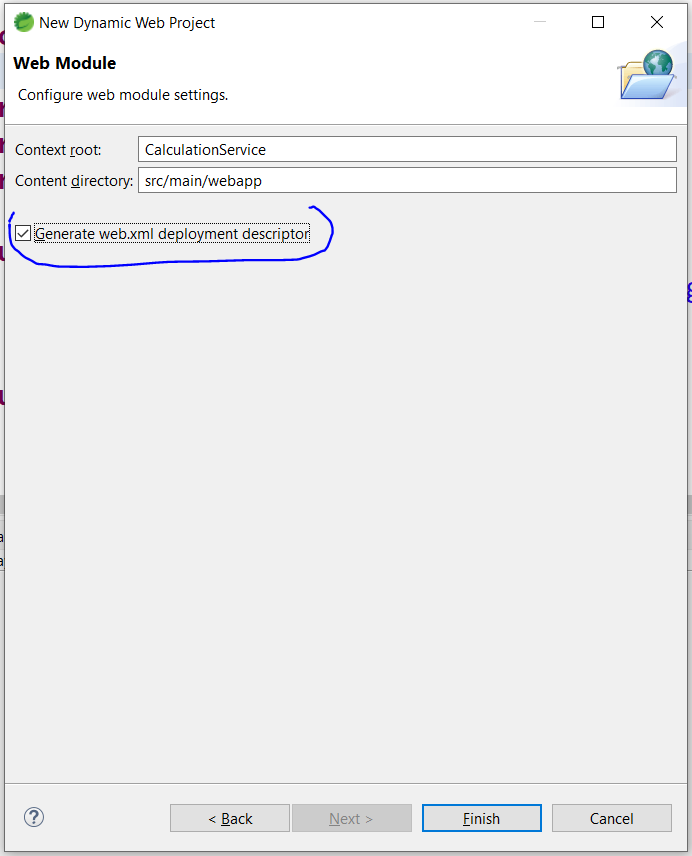
Step 4 : Click Finish. The dynamic web project should be created like below
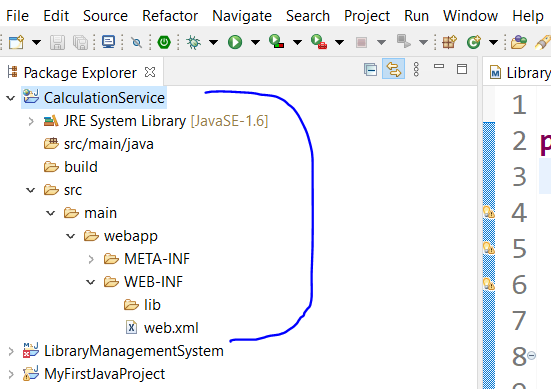
Step 5 : Create a Model Class called Operand under com.sks package
package com.sks; public class Operand < private int num1; private int num2; public int getNum1() < return num1; >public void setNum1(int num1) < this.num1 = num1; >public int getNum2() < return num2; >public void setNum2(int num2) < this.num2 = num2; >@Override public String toString() < return "Operand [num1=" + num1 + ", num2=" + num2 + "]"; >>
Step 6 : Create an interface CalculationService. It will have operations like sum, multiplication
package com.sks; public interface CalculationService
Step 7 : Create its implementation class CalculationServiceImpl. It implements CalculationService interface
package com.sks; public class CalculationServiceImpl implements CalculationService < @Override public int sum(Operand op) < return op.getNum1() + op.getNum2(); >@Override public int multiplication(Operand op) < return op.getNum1() * op.getNum2(); >>
Now, our dynamic web project is ready with some logic. Next step is to create a web service from it.
Step 8 : Create a new project in STS/eclipse and choose ‘Web Service’ under ‘Web Services’ option as shown below
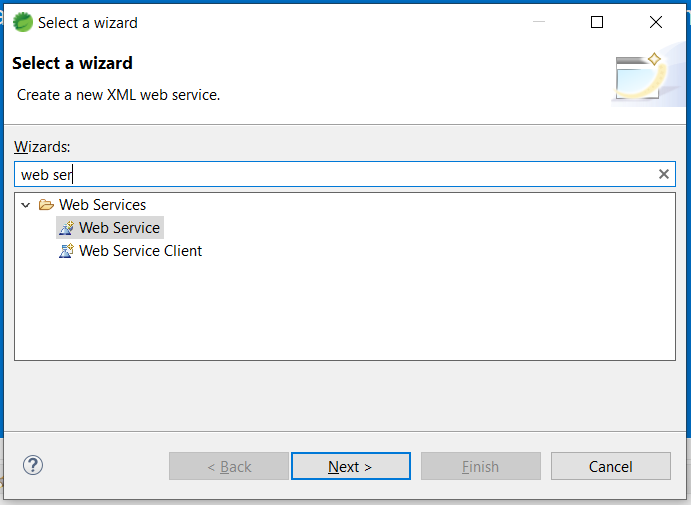
Step 9 : Now, click on Next and verify the details on the next screen. Make sure Web Service Type is selected as Bottom Up Java Bean Web Service.
Also, select Service implementation as our class CalculationServiceImpl. Also drag the Configuration bar to the maximum on the left hand side. It should be upto ‘Test Service’.
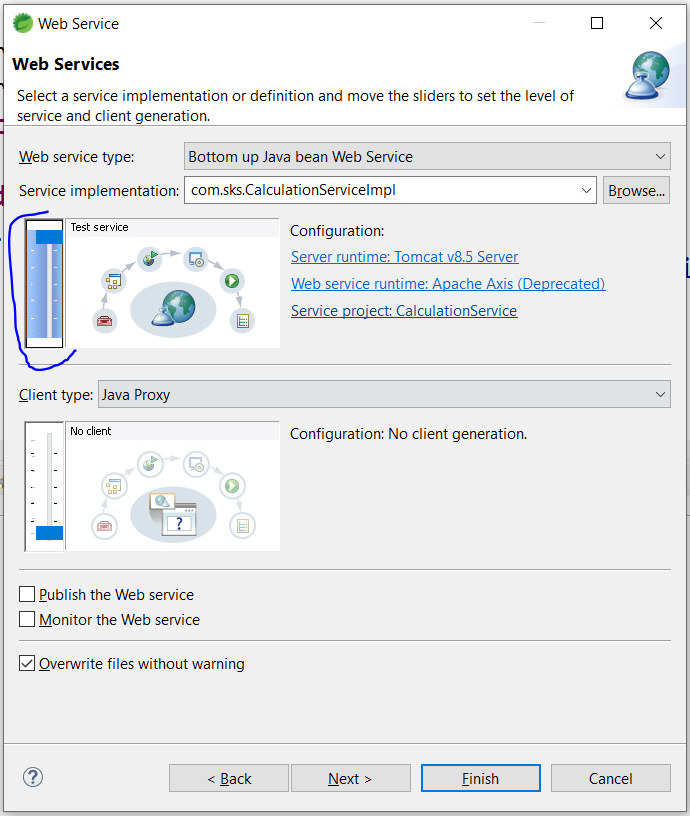
Step 10 : On the next screen, we can select which all methods we want to expose as web service. For this example, we will choose both
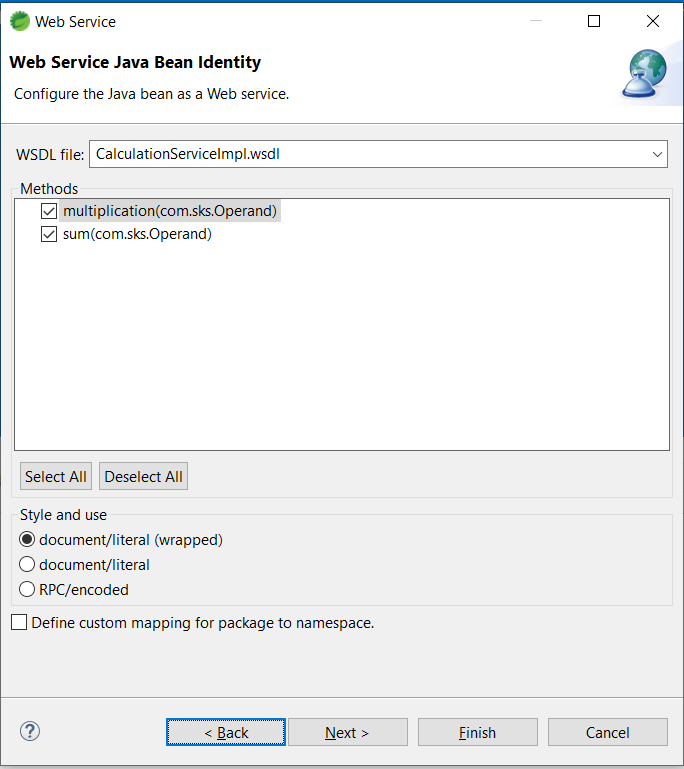
Step 11 : Click on Next. And cick on Start server button. Once it is started, click on Next button.
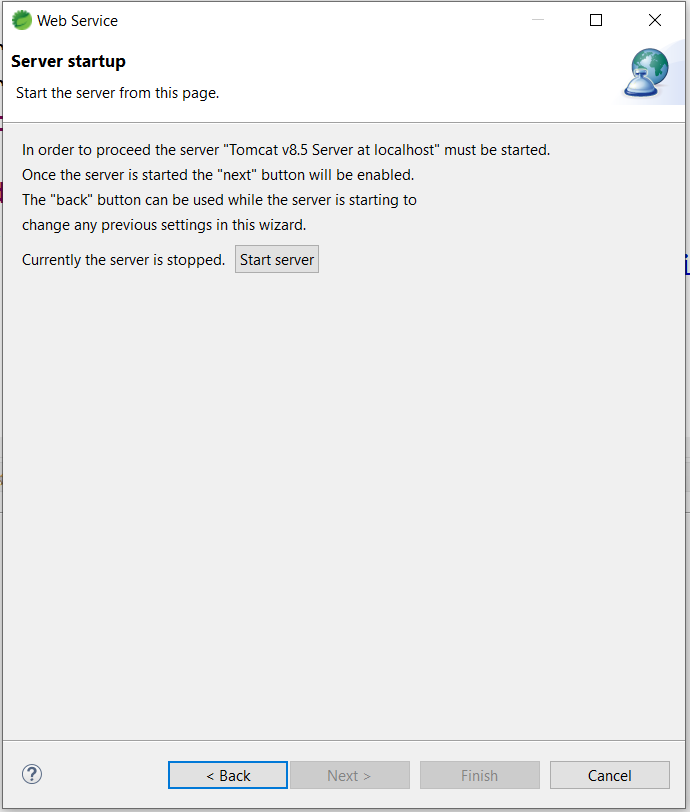
Step 12 : Below screen will appear. Test facility is selected as Web Services Explorer. Click on the Launch button.
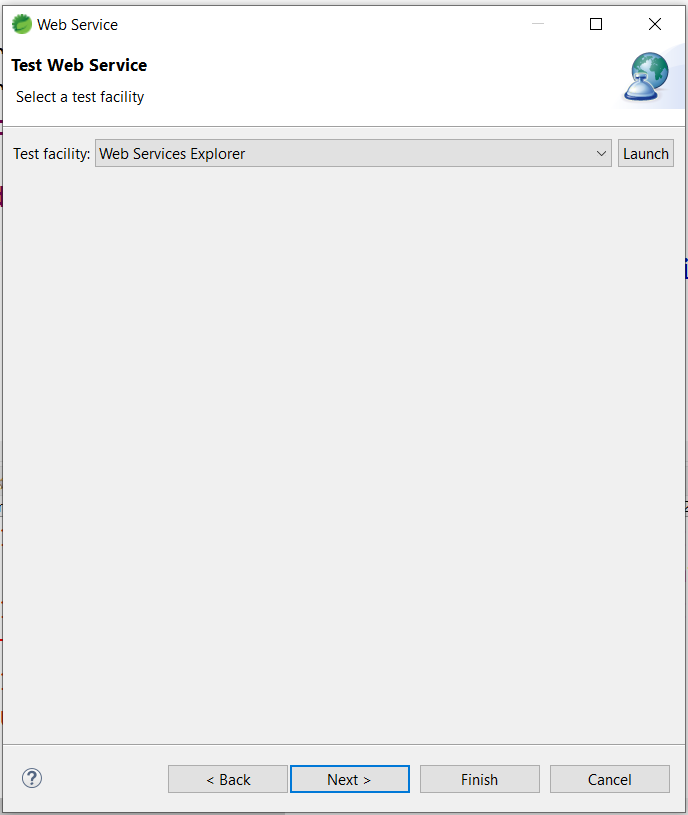
Step 12 : Web Services Explorer will open in a new window. It will look like below. Don’t do anything here and move to the next step.
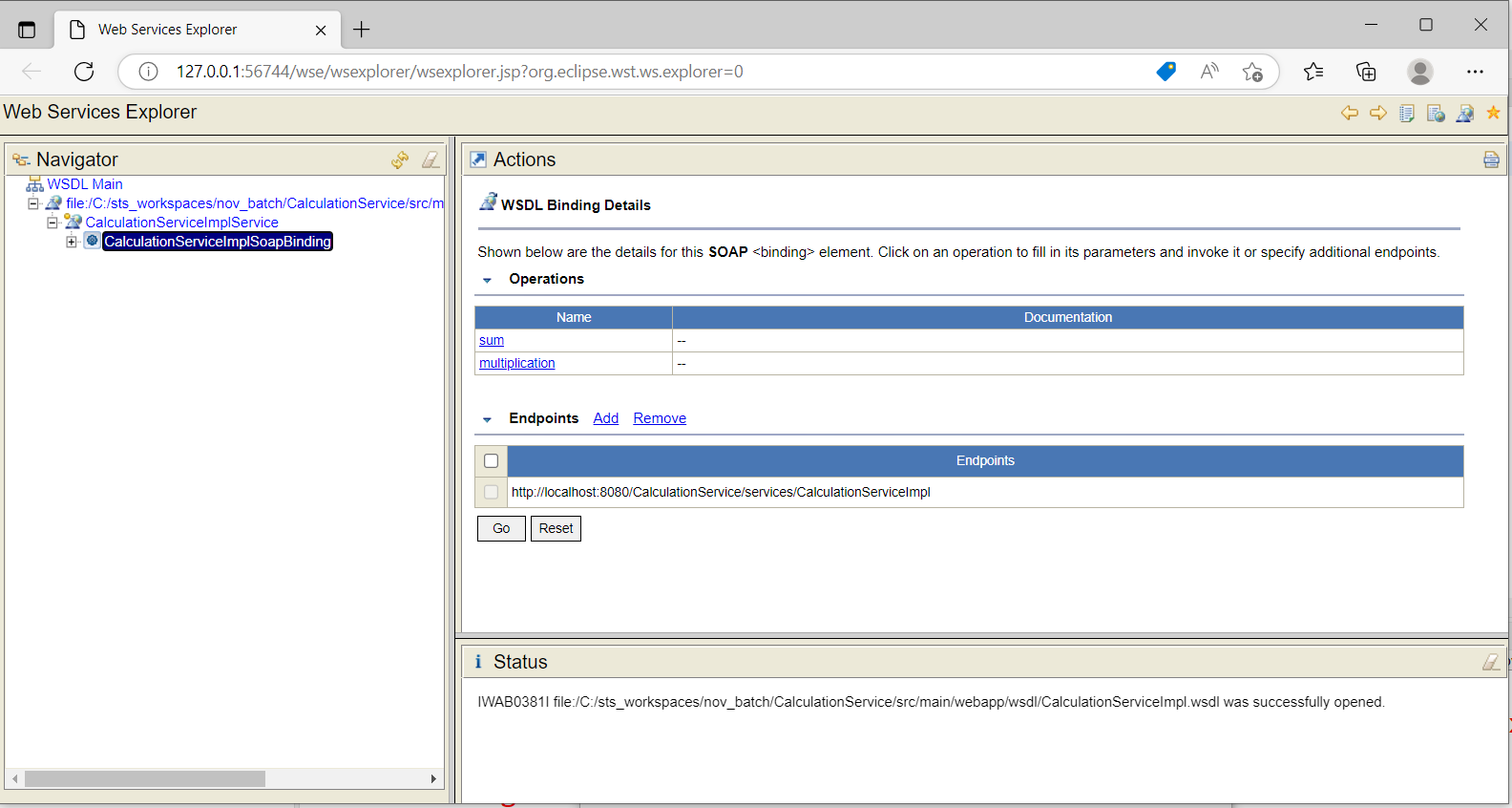
Step 13 : On the Eclipse, click on the Next button in the below screen
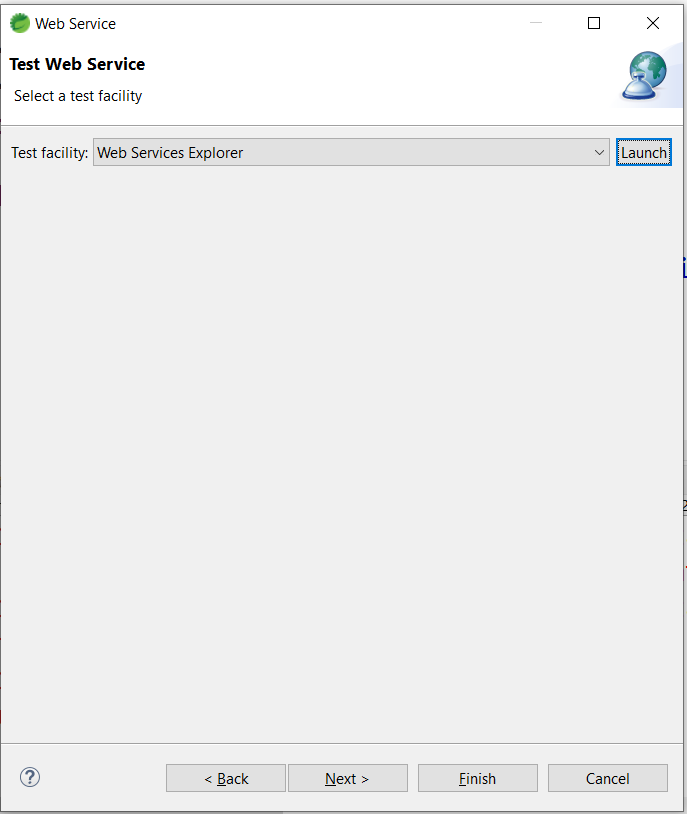
Step 14 : The below screen will appear. You don’t have to do anything on this screen. Just click on finish.
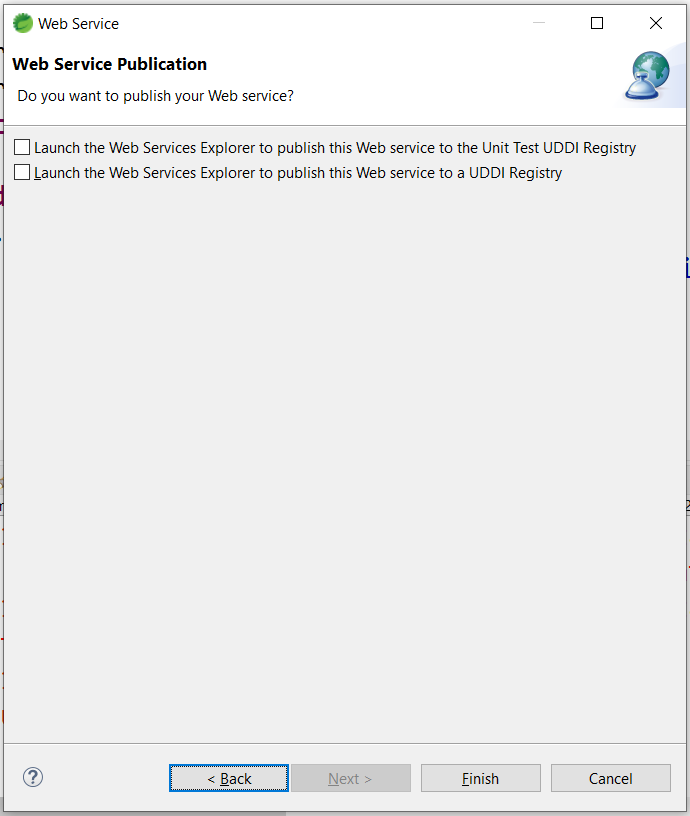
Step 15 : Go back to Web Services Explorer screen and click on sum as highlighted
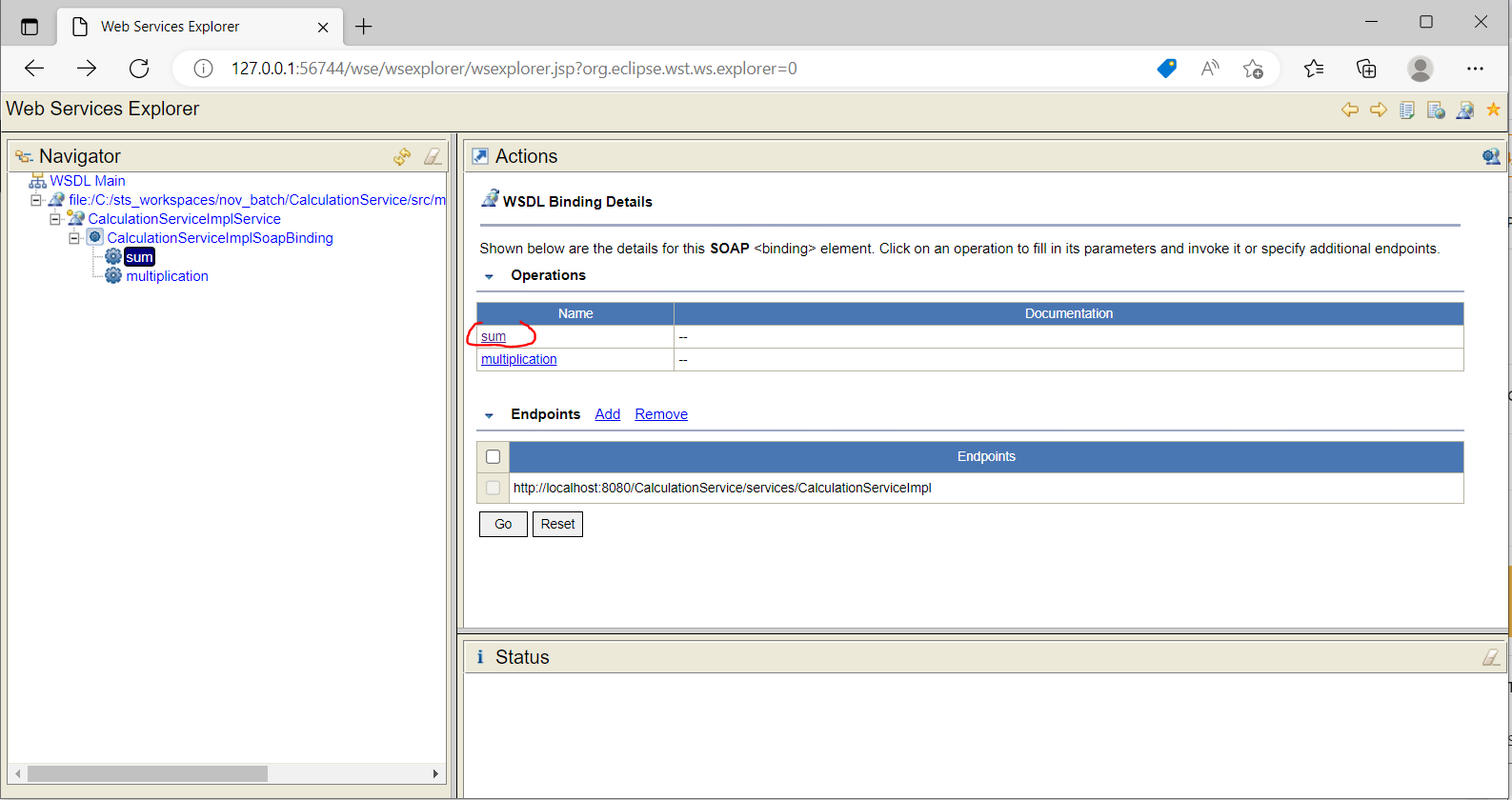
Step 16 : Give values in the num1 and num2 boxes and click Go button
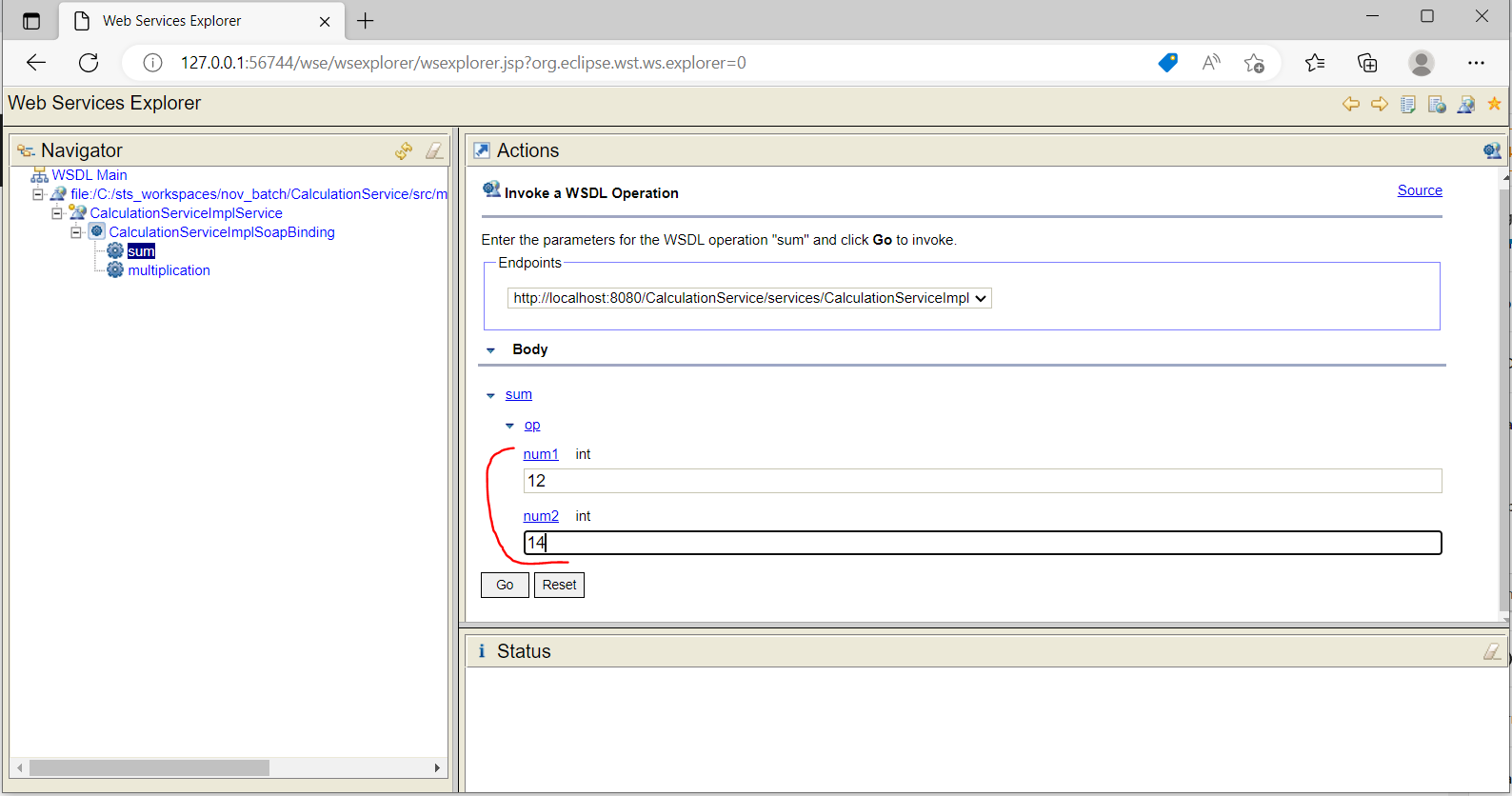
Step 17 : The result 26 can be seen under Status
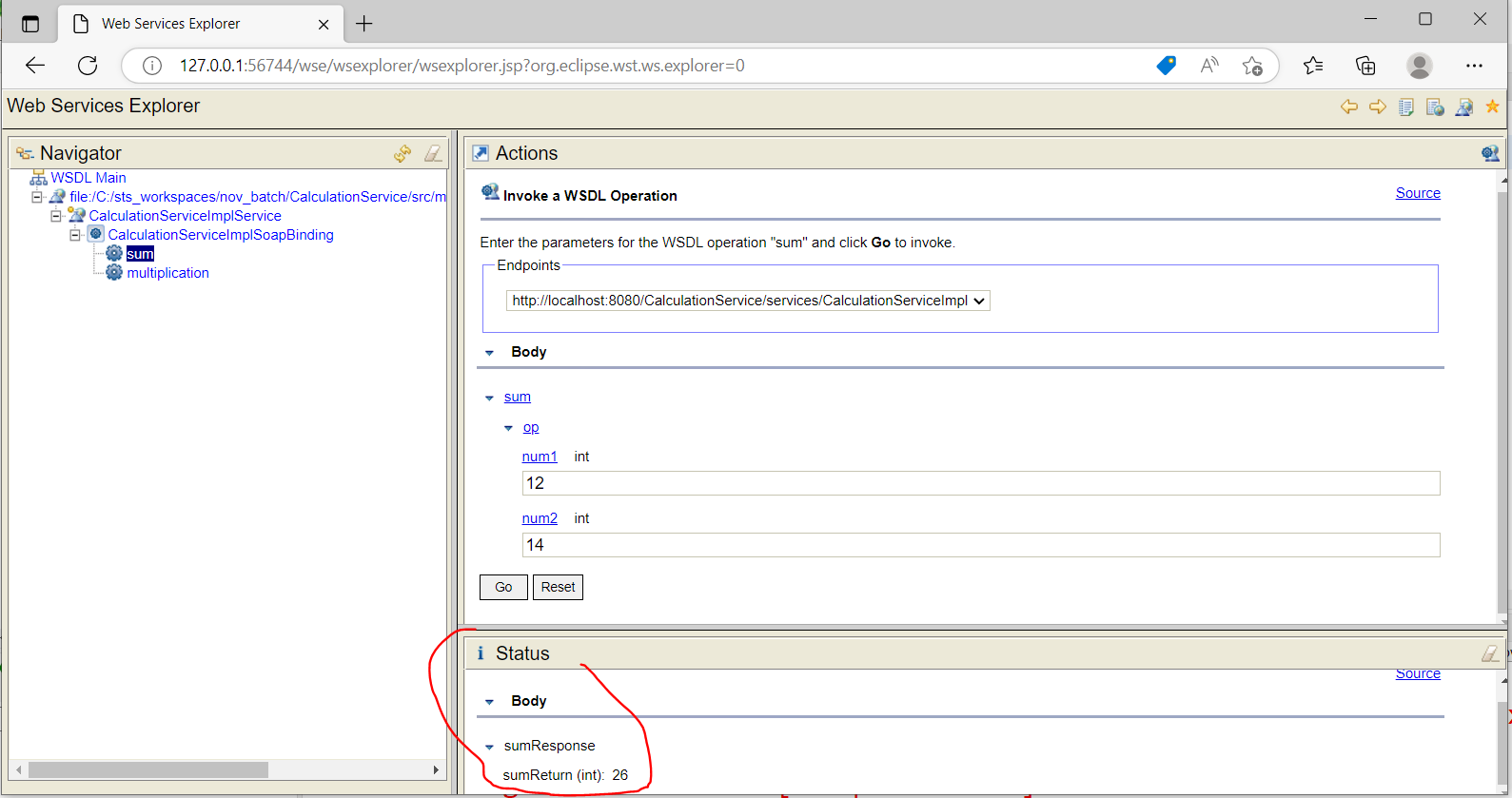
Step 18 : To check the soap generated request and response, click on the source as shown below
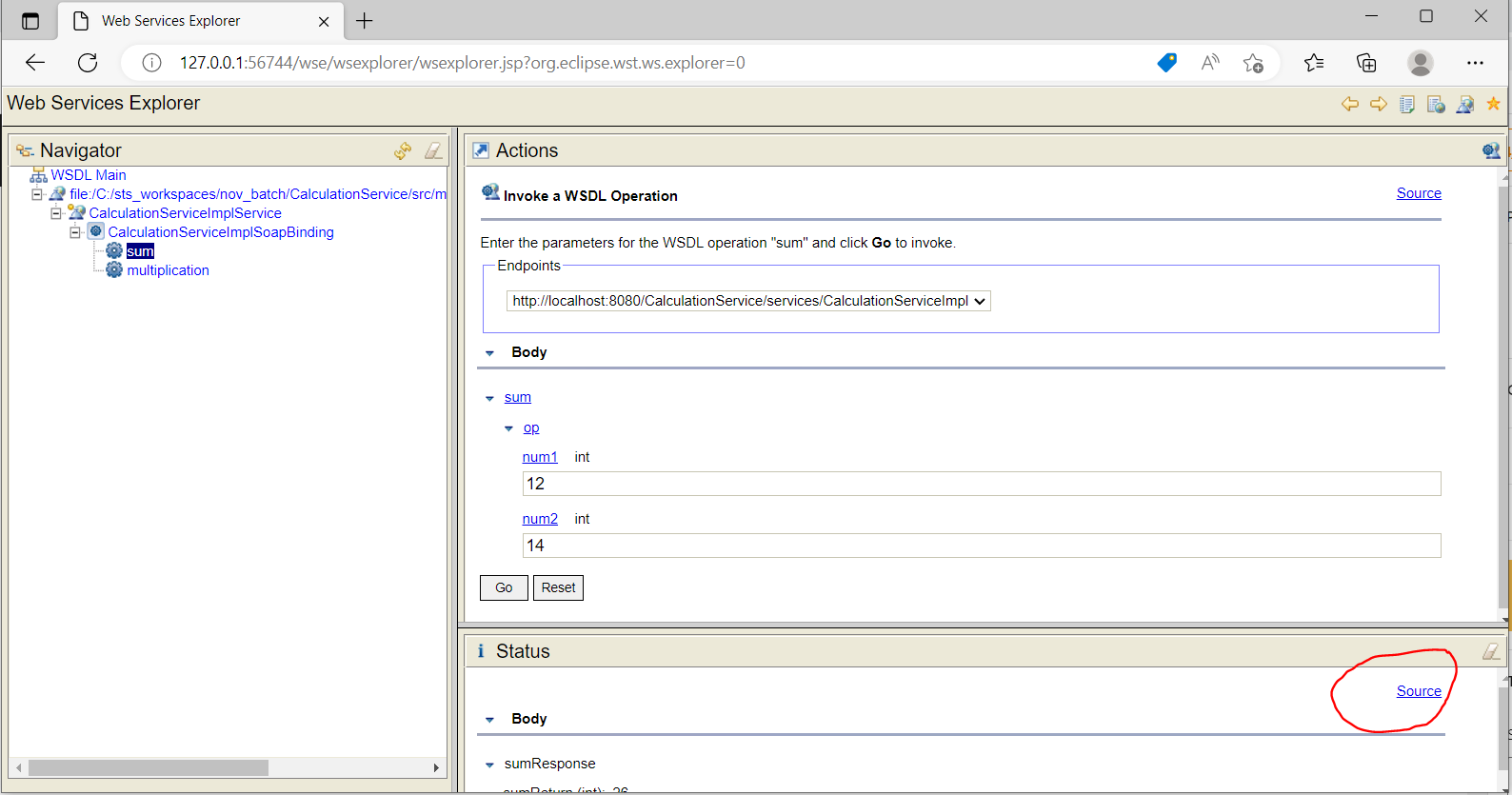
SOAP request
SOAP request for this method is as below
12 14
SOAP response
WSDL File
The WSDL file can be seen under the webapp folder as shown below
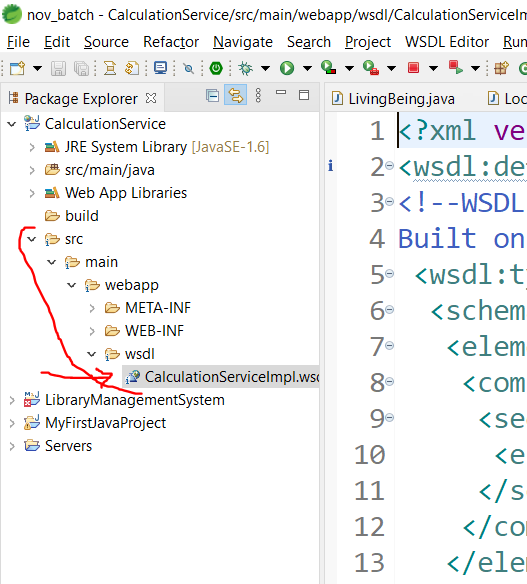
Below is the content of wsdl file
- Home
- Java
- Java Fundamentals
- What is Java
- History of Java
- Java Version History
- Local Environment Set-up
- First Java Program
- How to set ‘Path’ env variable
- JDK, JRE, and JVM
- Object Oriented Programming
- Java Data Types
- Classes in Java
- Objects in Java
- Interfaces in java
- Class attributes and methods
- Methods In Java
- Variables and Constants in Java
- Java packages and Imports
- Access Modifiers In Java
- Java Operators
- Constructors in Java
- Control Statements – If else
- Control Statements – Loops
- Arrays in Java
- Interfaces in Java
- Marker Interfaces
- Abstract classes in Java
- Difference Between Interfaces and Abstract Classes
- Inheritance
- Run Time Polymorphism vs Compile Time polymorphism
- Method Overloading in Java
- Auto Type Promotion in Overloading
- Method Overriding in Java
- Instance initializer Block
- Static Block
- instanceof operator
- Super Keyword
- Final keyword
- Java Exception Types
- Handle Exceptions using Try-Catch Block
- Finally block
- Checked Exception Example
- Nested try Blocks
- Throw Keyword
- Throws Keyword
- Exceptions and overriding
- Java custom exceptions
- String Introduction
- String Constant Pool vs HEAP
- How to compare Strings In Java
- Strings are immutable
- String Methods
- StringBuffer
- StringBuilder
- StringTokenizer Class
- toString() method
- Custom Immutable Classes
- File Handling In Java
- Creating File in Java
- Reading File in Java
- Deleting File in Java
- File IO using FileReader and FileWriter
- Collections Framework In Java
- List
- ArrayList in Java
- LinkedList in Java
- Vector in Java
- Stack in Java
- Sorting ArrayList With Comparable
- Sorting ArrayList using Comparator
- How to sort an Arraylist in java
- How to Sort a HashSet in Java
- Comparable vs Comparator
- HashSet in java
- LinkedHashSet in Java
- TreeSet in Java
- HashSet of Custom Objects in Java
- Queue and PriorityQueue
- Deque and ArrayDeque in Java
- HashMap in Java
- How to iterate over a HashMap in java
- LinkedHashMap in Java
- TreeMap in java
- HashMap with custom keys
- Equals and HashCode Contract
- How HashMap works internally
- EnumSet and EnumMap
- Hashtable in Java
- Concurrent Collections in Java
- ConcurrentHashMap in Java
- CopyOnWriteArrayList
- Multithreading
- Multithreading
- Creating first java thread
- Thread Lifecycle
- Start and Run method
- Join Method
- Thread.sleep() method
- Naming a thread
- Multiple tasks by multiple threads
- Thread Priorities
- Thread Pool / Group
- Daemon Thread
- Thread synchronization in java
- Difference between join method and synchronized method
- Synchronized methods
- Synchronized Block in java
- Static synchronization
- Wait, notify and notifyAll
- Deadlock and Livelock
- How to interrupt a thread
- How to stop a thread
- Java 8 Features
- Java 8 Features
- Default Method and Static Method in Interface
- How to solve diamond problem with java 8 default method
- Functional Interfaces
- Java Lambda Expressions
- Method references, Double Colon (::) Operator
- Java 8 Stream API
- Try With Resources
- Catch Multiple Exceptions
- Servlet API
- What is Servlet
- Servlet Life Cycle
- GenericServlet and HttpServlet
- Hello Servlet Example using Maven
- RequestDispatcher
- SendRedirect method
- ServletConfig Object
- ServletContext Object
- Session Tracking in Servlets
- URL Rewriting
- Cookies in Servlets
- Hidden form field
- HttpSession in Servlet
- JSP Introduction
- JSP Life Cycle
- First JSP application
- JSP Scripting Elements
- Scriptlet Tag
- Expression Tag
- Declaration Tag
- out Implicit Object
- request Implicit Object
- response Implicit Object
- config Implicit Object
- application Implicit Object
- session Implicit Object
- pageContext Implicit Object
- page Implicit Object
- exception Implicit Object
- JSP Page Directive
- JSP Include Directive
- JSP Taglib Directive
- Spring-Introduction
- First Spring Application
- Setter Injection
- Constructor injection
- Spring annotations
- Autowiring
- @Autowired Annotation Spring
- Spring JdbcTemplate
- Spring MVC
- Spring AOP
- Spring AOP Examples
- Download and configure Tomcat server
- Architecture
- Hibernate Example
- First Hibernate Application (using xml configuration)
- First Hibernate Application (using annotations)
- JPA/HB – annotations
- Hibernate Identifiers
- Hibernate Generator Classes
- Save vs saveOrUpdate vs persist in Hibernate
- Inheritance Mapping in Hibernate
- Inheritance Mapping using annotations
- Hibernate Mapping
- Hibernate One-to-One Mapping
- Hibernate one-to-many mapping
- Hiberate Many-to-Many Mapping
- Spring Boot Basics
- What is Spring Boot?
- First spring boot web application
- What is Maven?
- Spring Boot using STS
- Spring Boot Starters
- Spring boot application properties file
- Spring boot annotations
- @RequestParam
- Spring Boot Actuator
- RESTful microservice using Springboot
- Spring boot web application + thymeleaf
- Spring Boot + MVC + JSP
- Form validation with Springboot validators
- How to Generate SSH Key Pair on Windows Using the PuTTYgen
- Spring boot OAuth2
- Spring Boot + Spring Security + OAuth2
- Spring Boot Security With JWT
- Spring Boot + Junit5
- Spring Boot + junit5 + Mockito
- How to set up Oracle 19c database on local
- Set up MySQL database on Windows
- SQL Server Installation
- JDBC Connectivity Example with MySQL
- JDBC Connectivity Example with SQL Server
- JDBC Prepared Statement
- Call stored procedure in java
- SOAP Introduction
- SOAP Building Blocks
- SOAP Request Response
- SOAP Communication Model
- Create SOAP Web Service In Java Using Eclipse
- Create SOAP web service with Axis2 and maven in eclipse
- WSDL
- Generate Client From WSDL
- Difference between SOAP vs REST
- SOAP webservice basic authentication
- HTML Tutorial
- HTML, CSS Code Editor
- Blog
- Programs
- Simple Calculator in java
- Java console based gaming app
- Docker Java Tutorial
- Dockerize Spring Boot App
- Update Docker Image and Container
- Setup Kubernetes
- Docker Examples